Wavesurfer.js
Wavesurfer is a javascript library to analyze audio files and create an audio spectrum. It’s very similar to the ones that you can find on soundcloud and other music streaming sites. Wavesurfer also comes with its own audio player, so no additional HTML5 audio player is required. This javascript library is a very cool way to visualize audio files; especially if you a music streaming app or any other app that has an audio player associated with it.
Wavesurfer: https://wavesurfer-js.org

Audio Files App
To test wavesurfer, I created a basic web app, that allows users to upload audio files and then stream them on the fly. I used active storage to handle file uploads, it has a “audiofile” model with and attachment of “audio_data”. Since this app was just for testing, so i didn’t add any file validations, direct uploads or anything of that sort. I kept everything very simple and went just with the barebones. If i wanted to make this app for production, i would have added file validations, direct uploads and all of those other features as well.
Adding Wavesurfer assets
There are a number of ways to serve static assets to your rails app, i wanted to do it quickly so, i added them directly from wavesurfer’s CDN to my audio files’ show page.
#File: app/views/audiofiles/show.html.erb
<!--Other elements-->
<script src="https://unpkg.com/wavesurfer.js"></script>
<!--Other elements-->
Then i created a container, where the waveform will appear.
#File: app/views/audiofiles/show.html.erb
<!--Other elements-->
<script src="https://unpkg.com/wavesurfer.js"></script>
<div id="waveform"></div>
<!--Other elements-->
I could also customize the waveform like this, or i can leave it as default.
#File: app/views/audiofiles/show.html.erb
<script>
var wavesurfer = WaveSurfer.create({
container: '#waveform',
waveColor: 'violet',
progressColor: 'purple'
});
</script>
After that, i can finally load the source audio files like this.
#File: app/views/audiofiles/show.html.erb
<script>
wavesurfer.load('<%= rails_blob_url(@audiofile.audio_data) %>');
</script>
The end result
Finally after customizing a bit and also after adding the basic controls ,this is what the end result looks like.
#File: app/views/audiofiles/show.html.erb
<!--Other elements-->
<script src="https://unpkg.com/wavesurfer.js"></script>
<div id="waveform"></div>
<script>
var wavesurfer = WaveSurfer.create({
container: '#waveform',
waveColor: 'white',
progressColor: 'black',
cursorColor: 'black',
barWidth: 3,
barRadius: 3,
cursorWidth: 1,
height: 200,
barGap: 3
});
</script>
<script> wavesurfer.load('<%= rails_blob_url(@audiofile.audio_data) %>'); </script>
<div class="controls">
<button class="btn btn-primary" onclick="wavesurfer.skipBackward()"><i class="fa fa-step-backward"></i>Backward</button>
<button class="btn btn-primary" onclick="wavesurfer.playPause()"><i class="fa fa-play"></i>Play/<i class="fa fa-pause"></i>Pause</button>
<button class="btn btn-primary" onclick="wavesurfer.skipForward()"><i class="fa fa-step-forward"></i>Forward</button>
<button class="btn btn-primary" onclick="wavesurfer.toggleMute()"><i class="fa fa-volume-off"></i>Toggle Mute</button>
</div>
<!--Other elements-->
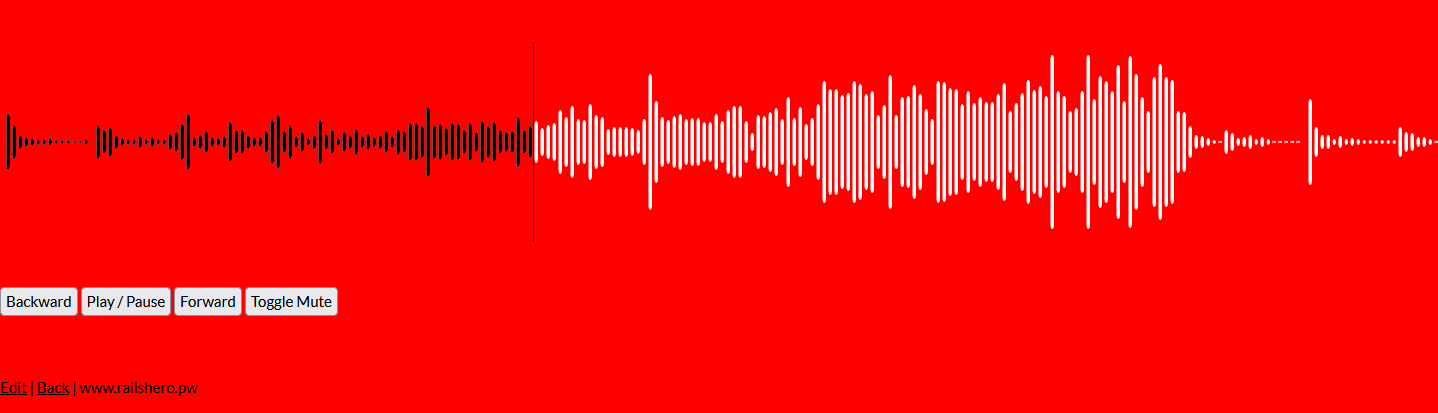
Final Thoughts
Wavesurfer can be customized in a number of ways; you can also add many plugins, plugins like spectrogram plugin, playlist plugin, etc. Better controls can also be added to look a lot nicer. Overall, i will say that this is a really great javascript library, that does the job with a very minimal setup process. That’s all folks please don’t forget to checkout wavesurfer docs, before using it. And have a nice day
Wavesurferdocs: https://wavesurfer-js.org/docs